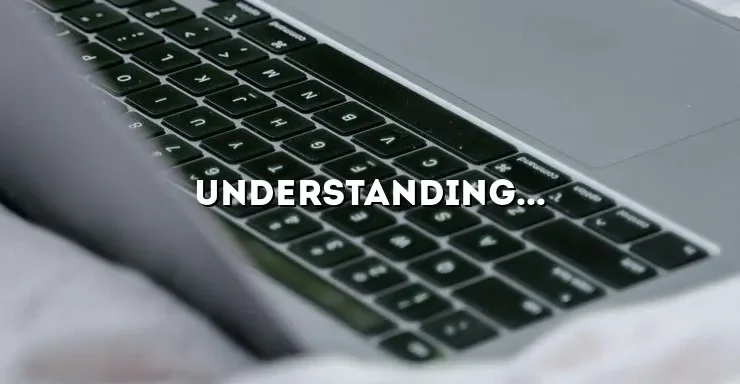
As a fundamental concept in computer science, the term “as” plays a crucial role in various programming languages and is often used to perform a wide range of operations. Whether you are a beginner or an experienced programmer, having a deep understanding of “as” can greatly enhance your ability to write efficient and error-free code. In this blog article, we will delve into the intricacies of “as” in computer science, exploring its different uses and providing comprehensive explanations and examples along the way.
First and foremost, “as” is frequently used in computer science as a type-casting operator. This means that it allows you to convert one data type into another. Understanding how to properly utilize “as” for type casting is essential for ensuring the compatibility and integrity of your code. From converting integers to strings to casting objects into specific classes, we will explore the various scenarios in which “as” can be utilized for type casting and provide practical examples to solidify your understanding.
Type Casting with “as”
In this section, we will dive into the details of type casting using the “as” operator. Type casting is the process of converting one data type into another, and “as” provides a powerful mechanism for performing this conversion. We will start by discussing the basic syntax and usage of “as” for type casting. Then, we will explore different scenarios where “as” can be employed, such as converting numeric types, casting to derived classes, and transforming objects into interfaces.
Basic Syntax and Usage
The basic syntax for type casting with “as” is as follows: variable as type
. Here, variable
is the variable you want to convert, and type
is the target data type you want to cast the variable to. The “as” operator attempts to perform the conversion and returns either the casted value or null
if the conversion fails. It is important to note that “as” can only be used for reference types and nullable value types.
Let’s consider an example to illustrate the usage of “as” for type casting:
int number = 10;object obj = number as object;
In this example, we have an integer variable number
, and we want to cast it to an object
. By using the “as” operator, we can perform the type casting. The variable obj
will now hold the value of number
as an object
. This allows us to work with the variable as an object
and access its properties and methods that are specific to the object
class.
Converting Numeric Types
One common use case for type casting with “as” is converting between different numeric types. For example, you may need to convert an integer to a floating-point number or convert a double to a long. The “as” operator can be used to perform these conversions safely and efficiently.
Let’s consider an example where we have an integer variable number
and we want to convert it to a double:
int number = 10;double convertedNumber = number as double;
Since “as” can only be used for reference types and nullable value types, the above code will not compile successfully. In this case, we need to use other operators or methods provided by the programming language to perform the desired conversion. For example, in C#, we can use the Convert.ToDouble()
method to convert the integer to a double:
int number = 10;double convertedNumber = Convert.ToDouble(number);
This code will successfully convert the integer number
to a double and assign the result to the variable convertedNumber
.
Casting to Derived Classes
Inheritance is a fundamental concept in object-oriented programming, and “as” can be used to cast objects to their derived classes. This allows you to access the specific properties and methods defined in the derived class that are not present in the base class.
Let’s consider an example where we have a base class Animal
and a derived class Cat
:
Animal animal = new Cat();Cat cat = animal as Cat;
In this example, we create an instance of the derived class Cat
and assign it to a variable of the base class Animal
. By using the “as” operator, we can safely cast the animal
variable to a Cat
. The variable cat
will now reference the same object but with the additional capabilities provided by the Cat
class.
Transforming Objects into Interfaces
Interfaces define a set of methods and properties that a class must implement. By using the “as” operator, you can cast an object to an interface and access the functionality defined by that interface.
Let’s consider an example where we have a class Rectangle
that implements the IShape
interface:
Rectangle rectangle = new Rectangle();IShape shape = rectangle as IShape;
In this example, we create an instance of the Rectangle
class and assign it to a variable of type IShape
, which is the interface implemented by the Rectangle
class. By using the “as” operator, we can safely cast the rectangle
variable to an IShape
. The variable shape
will now reference the same object but allow us to interact with it using the methods defined in the IShape
interface.
Exception Handling with “as”
Exception handling is a critical aspect of writing robust code, and “as” can greatly enhance your ability to handle exceptions gracefully. In this section, we will explore the different ways “as” can be utilized in exception handling, including catching specific exceptions and creating custom exception classes. We will also discuss the importance of proper exception handling and provide practical examples to demonstrate the power of “as” in this context.
Catching Specific Exceptions
When dealing with exceptions, it is often necessary to handle different types of exceptions in different ways. The “as” operator can be used in conjunction with the catch
statement to catch specific exceptions and perform appropriate actions based on the type of the caught exception.
Let’s consider an example where we have a method Divide
that divides two numbers:
public double Divide(double dividend, double divisor){try{return dividend / divisor;}catch (Exception ex){if (ex is DivideByZeroException){Console.WriteLine("Cannot divide by zero.");}else if (ex is OverflowException){Console.WriteLine("An overflow occurred.");}else{Console.WriteLine("An error occurred.");}return 0;}}
In this example, we attempt to divide the dividend
by the divisor
. If an exception occurs during the division, the catch
block will be executed. By using the “as” operator in conjunction with the is
keyword, we can check the type of the caught exception and perform different actions accordingly. In this case, if a DivideByZeroException
or an OverflowException
is caught, a specific message is displayed. Otherwise, a generic error message is printed.
Creating Custom Exception Classes
In addition to catching built-in exceptions, you can also create custom exception classes and use the “as” operator to catch and handle them separately. This allows you to define your own exception hierarchy and provide more granular error handling in your code.
Let’s consider an example where we have a custom exception class InvalidInputException
:
public class InvalidInputException : Exception{public InvalidInputException(string message) : base(message){}}public double Divide(double dividend, double divisor){try{if (divisor == 0){throw new InvalidInputException("Divisor cannot be zero.");}return dividend / divisor;}catch (InvalidInputException ex){Console.WriteLine(ex.Message);return 0;}catch (Exception ex){Console.WriteLine("An error occurred: " + ex.Message);return 0;}}
In this example, we have defined a custom exception class InvalidInputException
that inherits from the base Exception
class. In the Dividedivisor
is zero and throw an instance of the InvalidInputException
if it is. By using the “as” operator with the catch
statement, we can specifically catch and handle instances of the InvalidInputException
separately from other exceptions. This allows us to provide a custom error message for the invalid input case while still handling other exceptions with a generic error message.
“As” in Generic Programming
Generic programming is a powerful technique that allows for code reusability and flexibility. In this section, we will explore how “as” can be used in generic programming to ensure type safety and enable generic algorithms to work with a wide range of data types. We will discuss the syntactical aspects of using “as” in generic programming and provide examples to showcase its practical applications.
Using “as” with Generic Types
In generic programming, “as” can be used to cast a generic type parameter to a specific type. This is useful when you need to perform operations that are specific to a particular type within a generic algorithm.
Let’s consider an example where we have a generic class List<T>
and a method PrintLength
that prints the length of the elements in the list:
public class List<T>{private List<T> items;public void Add(T item){items.Add(item);}public void PrintLength(){foreach (var item in items){if (item is string str){Console.WriteLine($"Length of \"{str}\": {str.Length}");}else if (item is int number){Console.WriteLine($"Value of {number}: {number}");}else{Console.WriteLine("Unsupported type.");}}}}// UsageList<object> myList = new List<object>();myList.Add("Hello");myList.Add(42);myList.PrintLength();
In this example, we have a generic class List<T>
that contains a list of items of type T
. The Add
method allows adding items to the list, and the PrintLength
method prints the length of the elements in the list. By using the “as” operator in conjunction with the is
keyword, we can check the type of each item in the list and perform specific operations accordingly. In this case, if the item is a string, we print its length, and if it is an integer, we print its value. For unsupported types, we print a generic message.
Using “as” with Generic Interfaces
In addition to using “as” with generic types, you can also use it with generic interfaces. This allows you to cast a generic type parameter to a specific interface and access the members defined by that interface.
Let’s consider an example where we have a generic class Logger<T>
that logs messages and a generic interface ILoggable<T>
that defines the log method:
public interface ILoggable<T>{void Log(T message);}public class Logger<T> : ILoggable<T>{public void Log(T message){if (message is string str){Console.WriteLine($"Logging message: {str}");}else{Console.WriteLine("Unsupported message type.");}}}
// UsageLogger<string> stringLogger = new Logger<string>();stringLogger.Log("Hello, world!");
Logger<int> intLogger = new Logger<int>();intLogger.Log(42);
In this example, we have a generic interface ILoggable<T>
that defines a single method Log
. The generic class Logger<T>
implements this interface and provides a specific implementation for the Log
method. By using the “as” operator, we can check the type of the message parameter and perform specific logging operations accordingly. In this case, if the message is a string, we log it with a specific format, and for unsupported types, we print a generic message.
“As” in Database Querying
When working with databases, “as” can be a valuable tool for querying and retrieving specific data. In this section, we will explore how “as” can be used in SQL queries to perform operations such as aliasing column names and transforming data types. We will also discuss the limitations and best practices of using “as” in database querying to ensure efficient and accurate data retrieval.
Aliasing Column Names
In SQL, the “as” keyword can be used to alias column names in the result set of a query. This allows you to provide more meaningful or concise names for the returned columns, improving the readability of the query results.
Let’s consider an example where we have a table Employees
with columns FirstName
and LastName
:
SELECT FirstName as First, LastName as Last FROM Employees;
In this example, we use the “as” keyword to alias the FirstName
column as First
and the LastName
column as Last
. This means that the query result will have columns with the aliases First
and Last
instead of the original column names. This can make the query results more descriptive and easier to understand.
Transforming Data Types
The “as” keyword can also be used to transform data types in SQL queries. This can be useful when you need to perform calculations or comparisons with columns of different data types.
Let’s consider an example where we have a table Orders
with columns OrderDate
of type datetime
and TotalAmount
of type decimal
:
SELECT OrderDate as Date, TotalAmount as Amount, CAST(TotalAmount as int) as RoundedAmount FROM Orders;
In this example, we use the “as” keyword to alias the OrderDate
column as Date
and the TotalAmount
column as Amount
. Additionally, we use the CAST
function to transform the TotalAmount
column into an integer and alias it as RoundedAmount
. This allows us to perform calculations or comparisons with the rounded amount, if needed.
Performance Considerations with “as”
While “as” offers flexibility and convenience in many scenarios, it is important to consider its impact on performance. In this section, we will delve into the performance implications of using “as” extensively, discussing potential bottlenecks and suggesting optimization techniques. By understanding the performance considerations associated with “as,” you will be able to write code that is not only correct but also performs efficiently.
Potential Performance Bottlenecks
Using the “as” operator extensively can introduce performance bottlenecks in your code. The type casting operation performed by “as” involves runtime checks and conversions, which can be costly in terms of execution time and memory usage.
One potential performance bottleneck is the repeated use of “as” in a loop. If you have a loop that performs type casting on each iteration, the overhead of the type checks and conversions can accumulate, resulting in a significant performance impact. In such cases, it is advisable to consider alternative approaches or optimizations to minimize the use of “as” within the loop.
Optimization Techniques
To optimize the performance of code that uses the “as” operator, there are several techniques you can employ:
1. Minimize the Use of “as”
Review your code and identify areas where the use of “as” can be minimized or avoided altogether. Consider whether alternative approaches, such as using specific type checks or leveraging polymorphism, can achieve the same result without the need for type casting.
2. Cache the Casted Value
If you find yourself repeatedly casting a variable to the same type within a particular scope, consider caching the casted value to avoid multiple type checks and conversions. This can be especially beneficial in scenarios where the type casting operation is expensive or involves complex calculations.
3. Use Explicit Type Casting
In some cases, using explicit type casting operators, such as (type)variable
, instead of “as” can offer better performance. Explicit type casting assumes that the conversion will always succeed, so it doesn’t perform runtime checks. However, it can result in runtime exceptions if the conversion fails.
4. Profile and Benchmark
To accurately assess the performance impact of using “as” in your code, it is important to profile and benchmark your application. Profiling tools can help you identify hotspots where the use of “as” is causing performance degradation. By measuring the execution time and memory usage of different parts of your codebase, you can pinpoint areas that require optimization and make informed decisions on how to improve performance.5. Consider Compiler Optimization
Depending on the programming language and compiler you are using, there may be compiler optimizations in place to optimize the use of “as” operator. Compiler optimizations can analyze your code and automatically apply optimizations to reduce the overhead of type casting. Keeping your compiler up to date and leveraging compiler optimization flags can help improve the performance of your code.
Trade-offs and Best Practices
While it is important to be mindful of the performance implications of using “as,” it is equally crucial to maintain code clarity and readability. Striking the right balance between performance and maintainability is a key consideration in software development.
Here are some best practices to keep in mind when using “as” in your code:
1. Use “as” When Necessary
Only use the “as” operator when it is necessary for type casting. Consider alternative approaches, such as polymorphism or generics, to achieve the desired functionality without the need for type casting.
2. Use “as” Judiciously in Performance-Critical Sections
If you have identified performance-critical sections in your code, carefully evaluate the use of “as” and consider optimizing or refactoring those sections to minimize the overhead of type casting.
3. Profile and Benchmark
Regularly profile and benchmark your code to identify areas that require optimization. This will help you make informed decisions on how to improve the performance of your application without sacrificing maintainability.
4. Document and Comment
When using “as” in your code, document the rationale behind its usage and any performance considerations associated with it. This will help other developers understand the purpose and implications of the type casting operations.
5. Keep Abstractions Clean
When designing abstractions or APIs, consider the impact of type casting operations on the users of those abstractions. Strive to provide clear and intuitive interfaces that minimize the need for type casting and promote type safety.
By following these best practices and being mindful of the performance implications, you can effectively use the “as” operator while maintaining code performance and readability.
In conclusion, the concept of “as” in computer science is multifaceted and plays a crucial role in various programming scenarios. From type casting to exception handling, generic programming, database querying, and performance considerations, “as” offers a wide range of functionalities that can greatly enhance your programming skills. By gaining a comprehensive understanding of “as,” you will be equipped with a powerful tool to write efficient, error-free, and flexible code in various programming languages.